Document for Salesforce apex
1.Math expression eval object initialization
Afe_Evaluator expr = new Afe_Evaluator('IF(abc>1,"passed","fail")');
or using default constructor + setExpression() method:
Afe_Evaluator expr = new Afe_Evaluator(); expr.setExpression('IF(abc>1,"passed","fail")');
2.Bind variable
Afe_Evaluator expr = new Afe_Evaluator('IF(abc>1,"passed","fail")'); expr.bind('abc',2);
3. Eval the expression
//Class initialization Afe_Evaluator expr = new Afe_Evaluator('IF(abc>1,"passed","fail")'); //Bind variable expr.bind('abc',2); //eval expr.eval(); // return "passed"
Result:
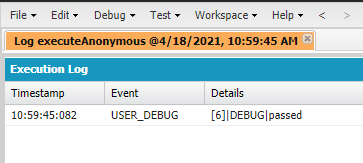
4. Multiple methods on one line
new Afe_Evaluator('IF(abc>1,"passed","fail")').bind('abc',2).eval();
5. Conditional statements
IF/ELSE condition:
//Class initialization Afe_Evaluator expr = new Afe_Evaluator('IF(OR(AND(abc>1, def<100),AND(abc < 1, abc > 0)),"passed","fail")'); //Bind variable expr.bind('abc',2); expr.bind('def',99); //eval expr.eval(); // return "passed"
SWITCH/CASE condition:
//Class initialization Afe_Evaluator expr = new Afe_Evaluator('SWITCH(abc,1,"apple",2,"mango",3,"banana","N/A")'); //Bind variable expr.bind('abc',2); System.debug(expr.eval()); //return "mango"
6. Set rounding mode and decimal precision
Use setScale() method to set number of digits to the right of the decimal point. Use setRoundingMode() method to set rounding mode. For salesforce apex language, you can use 5 mode as below:
- RoundingMode.CEILING
- RoundingMode.DOWN
- RoundingMode.FLOOR
- RoundingMode.HALF_DOWN
- RoundingMode.HALF_EVEN
- RoundingMode.HALF_UP
- RoundingMode.UP
Afe_Evaluator expr = new Afe_Evaluator('10/3'); //Set scale expr.setScale(2); //Set rounding mode expr.setRoundingMode(RoundingMode.UP); //eval System.debug(expr.eval()); // will return 3.34
7. Work with number expression
Example 1: Simple expression
Afe_Evaluator expr = new Afe_Evaluator('(a+b+c)/2'); expr.bind('a',2); expr.bind('b',3); expr.bind('c',16); expr.eval(); // return 10.5
Example 2: Complex expression
Afe_Evaluator expr = new Afe_Evaluator('(a^2+b^2+sqrt(c))%5'); expr.bind('a',2); expr.bind('b',3); expr.bind('c',16); expr.eval(); // return 2
Example 3: Min, max
Afe_Evaluator expr = new Afe_Evaluator('MIN(a,b,c)'); expr.bind('a',2); expr.bind('b',3); expr.bind('c',16); expr.eval(); // return 2
Example 4: Min, max with collection variable
Afe_Evaluator expr = new Afe_Evaluator('MAX(a)'); expr.bind('a',new List<Decimal>{10, 9.6, 135, 724.2, 6, -4}); expr.eval(); // return 724.2
Example 5: Sum, average
Afe_Evaluator expr = new Afe_Evaluator('SUM(a,b,c)'); expr.bind('a',2); expr.bind('b',3); expr.bind('c',16); expr.eval(); // return 21 expr.setExpression('AVERAGE(a,b,c)'); expr.eval(); // return 7
Example 6: Sum, average using collection variable
Afe_Evaluator expr = new Afe_Evaluator('SUM(a)'); expr.bind('a',new List<Decimal>{2, 3, 16}); expr.eval(); // return 21 expr.setExpression('AVERAGE(a)'); expr.eval(); // return 7
Example 7: Round, Floor, Ceiling
Afe_Evaluator expr = new Afe_Evaluator('ROUND(2.149, 1)'); System.debug(expr.eval()); //return 2.1
Afe_Evaluator expr = new Afe_Evaluator('FLOOR(2.149)'); System.debug(expr.eval()); //return 2
//Rounds 3.7 down to nearest multiple of 2 Afe_Evaluator expr = new Afe_Evaluator('FLOOR(3.7,2)'); System.debug(expr.eval()); //return 2
Afe_Evaluator expr = new Afe_Evaluator('CEILING(2.149)'); System.debug(expr.eval()); //return 3
//Rounds 1.5 up to the nearest multiple of 0.1 Afe_Evaluator expr = new Afe_Evaluator('CEILING(1.5, 0.1)'); System.debug(expr.eval()); //return 1.5
Example 8: Trigonometry calculation
Afe_Evaluator expr = new Afe_Evaluator('SIN(PI())^2+COS(PI())^2'); System.debug(expr.eval()); //return 1
Example 9: Unary operator
Afe_Evaluator expr = new Afe_Evaluator('3--2+--3-(-(++2))'); System.debug(expr.eval()); //return 10
8. Work with string
Example 1:
Afe_Evaluator expr = new Afe_Evaluator('IF(LEFT(abc,4)="2021","n/a","generation of " & LEFT(abc,4))'); expr.bind('abc','2022-01-20'); System.debug(expr.eval());
9. Work with datetime
Example 1: Calculate networkday
Afe_Evaluator expr = new Afe_Evaluator('NETWORKDAYS(DATEVALUE("2021-03-30"),DATEVALUE("2022-05-16"))'); System.debug(expr.eval()); // return 295
Afe_Evaluator expr = new Afe_Evaluator('NETWORKDAYS(startdate,enddate)'); expr.bind('startdate', Date.newInstance(2021,08,11)); expr.bind('enddate', Date.newInstance(2021,10,4)); System.debug(expr.eval()); // return 39
Example 2: Calculates the number of days, months, or years between two dates.
Afe_Evaluator expr = new Afe_Evaluator('DATEDIF(DATEVALUE("2021-01-31"),DATEVALUE("2029-08-29"),"Y")'); System.debug(expr.eval()); // return 8
Afe_Evaluator expr = new Afe_Evaluator('DATEDIF(DATEVALUE("2021-01-31"),DATEVALUE("2029-08-29"),"M")'); System.debug(expr.eval()); // return 102
Afe_Evaluator expr = new Afe_Evaluator('DATEDIF(DATEVALUE("2021-01-31"),DATEVALUE("2029-08-29"),"D")'); System.debug(expr.eval()); // return 3132
Afe_Evaluator expr = new Afe_Evaluator('DATEDIF(DATEVALUE("2021-01-31"),DATEVALUE("2029-08-29"),"MD")'); System.debug(expr.eval()); // return 29
Example: Substract, add datetime
Afe_Evaluator expr = new Afe_Evaluator('DATETIME(2021,2,28,15,50,0)-DATETIME(2021,2,21,23,0,0)'); System.debug(expr.eval()); // return 6.70139 (days)
Afe_Evaluator expr = new Afe_Evaluator('DATE(2021,2,28)-DATE(2021,2,21)'); System.debug(expr.eval()); // return 7 (days)
Afe_Evaluator expr = new Afe_Evaluator('DATE(2021,9,20)+3'); System.debug(expr.eval()); // return 2021-09-23
Afe_Evaluator expr = new Afe_Evaluator('TIME(10,10,0)+TIME(10,10,0)'); System.debug(expr.eval()); // return 20:20:00
10. Complex of number, string and datetime
11. Supported operators, constants and methods
Supported Operators
Operator | Description |
---|---|
+ | Additive operator / Unary plus / Concatenate string / Datetime addition |
& | Concatenate string |
– | Subtraction operator / Unary minus / Datetime subtraction |
* | Multiplication operator, can be omitted in front of an open bracket |
/ | Division operator |
% | Remainder operator (Modulo) |
^ | Power operator |
Supported conditional statements
Conditional statement | Description |
---|---|
IF(logical_condition, value_if_true, value_if_false) | Example:IF(2>1,"Pass","Fail") |
SWITCH(expression, val1,result1, [val2,result2], …, [default]) | Example:SWITCH(3+2,5,"Apple",7,"Mango",3,"Good","N/A") |
Supported logical and math functions
Function* | Description |
---|---|
AND(logical1, [logical2], …) | Determine if all conditions are TRUE |
OR(logical1, [logical2], …) | Determine if any conditions in a test are TRUE |
NOT(logical) | To confirm one value is not equal to another |
XOR(logical1, [logical2], …) | Exclusive OR function |
SUM(number1, [number2],…) | Return sum of numbers supplied |
AVERAGE(number1, [number2],…) | Return average of numbers supplied |
MIN(number1, [number2],…) | Return the smallest value from the numbers supplied |
MAX(number1, [number2],…) | Return the biggest value from the numbers supplied |
MOD(number, divisor) | Get remainder of two given numbers after division operator. |
ROUND(number, num_digits) | Returns the rounded approximation of given number using half-even rounding mode ( you can change to another rounding mode) |
FLOOR(number, significance) | Rounds a given number towards zero to the nearest multiple of a specified significance |
CEILING (number, significance) | Rounds a given number away from zero, to the nearest multiple of a given number |
POWER(number, power) | Returns the result of a number raised to a given power |
RAND() | Produces a random number between 0 and 1 |
SIN(number) | Returns the trigonometric sine of the angle given in radians |
SINH(number) | Returns the hyperbolic sine of a number |
ASIN(number) | Returns the arc sine of an angle, in the range of -pi/2 through pi/2 |
COS(number) | Returns the trigonometric cos of the angle given in radians |
COSH(number) | Returns the hyperbolic cos of a number |
ACOS(number) | Returns the arc cosine of an angle, in the range of 0.0 through pi |
TAN(number) | Returns the tangent of the angle given in radians |
TANH(number) | Returns the hyperbolic tangent of a number |
ATAN(number) | Returns the arc tangent of an angle given in radians |
ATAN2(x_number, y_number) | Returns the arctangent from x- and y-coordinates |
COT(number) | Returns the cotangent of an angle given in radians. |
COTH(number) | Returns the hyperbolic cotangent of a number |
SQRT(number) | Returns the correctly rounded positive square root of given number |
LN(number) | Returns the natural logarithm (base e) of given number |
LOG10(number) | Returns the logarithm (base 10) of given number |
EXP(number) | Returns e raised to the power of given number |
ABS(number) | Returns the absolute value of given number |
FACT(number) | Returns the factorial of a given number |
SEC(number) | Returns the secant of an angle given in radians |
CSC(number) | Returns the cosecant of an angle given in radians |
PI() | Return value of Pi |
RADIANS(degrees) | Convert degrees to radians |
DEGREES (radians) | Convert radians to degrees |
INT(number) | Returns the Integer value of given number |
Supported Constants
Constant | Description |
---|---|
e | The value of e |
PI | The value of PI |
TRUE | The boolean true value |
FALSE | The boolean false value |
NULL | The null value |
Supported text functions
Function | Description |
---|---|
LEFT(text, num_chars) | Extracts a given number of characters from the left side of a supplied text string |
RIGHT(text, num_chars) | Extracts a given number of characters from the right side of a supplied text string |
MID(text, start_num, num_chars) | Extracts a given number of characters from the middle of a supplied text string |
REVERSE(text) | Reverse a string |
ISNUMBER(text) | Check if a value is a number |
LOWER(text) | Converts all letters in the specified string to lowercase |
UPPER(text) | Converts all letters in the specified string to uppercase |
PROPER(text) | Capitalizes words given text string |
TRIM(text) | Removes extra spaces from text |
LEN(text) | Returns the length of a string/ text |
TEXT(value, [format_text]) | Convert a numeric value into a text string. You can use the TEXT function to embed formatted numbers inside text Example:
|
REPLACE(old_text, start_num, num_chars, new_text) | Replaces characters specified by location in a given text string with another text string |
SUBSTITUTE(text, old_text, new_text) | Replaces a set of characters with another |
FIND(find_text, within_text, [start_num]) | Returns the location of a substring in a string (case sensitive) |
SEARCH(find_text, within_text, [start_num]) | Returns the location of a substring in a string (case insensitive) |
CONCAT(text1, text2, text3,…) | Combines the text from multiple strings |
ISBLANK(text) | Returns TRUE when a given string is null or empty, otherwise return FALSE |
REPT(text, repeat_time) | Repeats characters a given number of times |
CHAR(char_code) | Return character from ascii code |
CODE(char) | Returns a ascii code of a character |
Supported date&time functions
Function | Description |
---|---|
DATE(year,month,day) | Constructs a Date from Integer representations of the year, month (1=Jan), and day |
DATEVALUE(date_string) | Constructs a Date from String representations of the year–month–day |
TIME(hour,min,second) | Constructs a Time instance from Integer representations of the hour, minute, and second |
SECOND(time_instance) SECOND(datetime_instance) | Returns the second component of a Time, Datetime |
MINUTE(time_instance) MINUTE(datetime_instance) | Returns the minute component of a Time, Datetime |
HOUR(time_instance) HOUR(datetime_instance) | Returns the hour component of a Time, Datetime |
DAY(date_instance) DAY(datetime_instance) | Returns the day component of a Date, Datetime |
MONTH(date_instance) MONTH(datetime_instance) | Returns the month component of a Date, Datetime |
YEAR(date_instance) YEAR(datetime_instance) | Returns the year component of a Date, Datetime |
NOW() | Returns the current Datetime based on a GMT calendar |
TODAY() | Returns the current date in the current user’s time zone. |
EDATE(start_date, months) | Add month to Date, Datime |
WEEKDAY(date_instance) | Returns the day of the week corresponding to a date. The day is given as an integer, ranging from 1 (Sunday) to 7 (Saturday) |
WEEKNUM(date_instance) | Returns the week number of a specific date. For example, the week containing January 1 is the first week of the year, and is numbered week 1 |
WORKDAY(start_date_instance, days) | Returns a number that represents a date that is the indicated number of working days before or after a date (the starting date). |
NETWORKDAYS(start_date_instance, end_date_instance) | Returns the number of whole workdays between two dates |
EOMONTH(start_date_instance, months) | Calculates the last day of the month after adding a specified number of months to a date, datetime |
DATEDIF(start_date, end_date, unit) | Calculates the number of days, months, or years between two dates. |
DAYS(end_date, start_date) | Returns the number of days between two dates |